leetcode 110 平衡二叉树
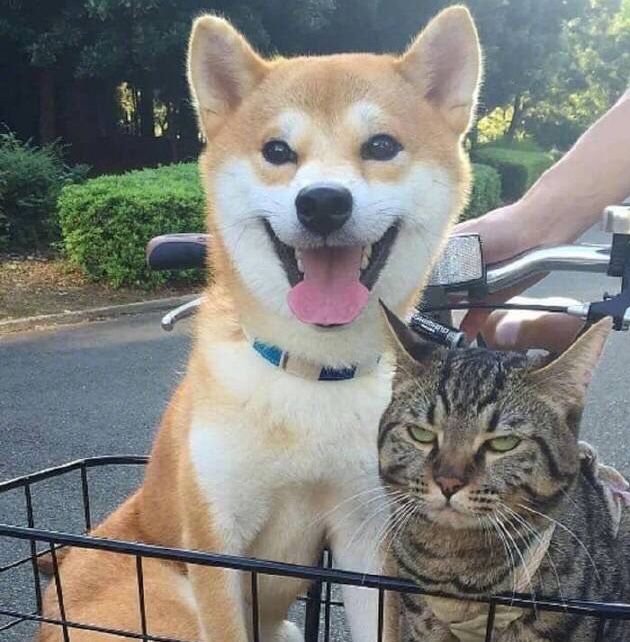
给定一个二叉树,判断它是否是高度平衡的二叉树。
本题中,一棵高度平衡二叉树定义为:
一个二叉树每个节点 的左右两个子树的高度差的绝对值不超过1。
示例 1:
给定二叉树 [3,9,20,null,null,15,7]
3
/ \
9 20
/ \
15 7
返回 true 。
示例 2:
给定二叉树 [1,2,2,3,3,null,null,4,4]
1
/ \
2 2
/ \
3 3
/ \
4 4
返回 false 。
递归的寻找当前节点的左子树和右子树的高度,如果高度差为不满足平衡关系,返回-1
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28# Definition for a binary tree node.
# class TreeNode(object):
# def __init__(self, x):
# self.val = x
# self.left = None
# self.right = None
class Solution(object):
def isBalanced(self, root):
"""
:type root: TreeNode
:rtype: bool
"""
def getDepth(root):
if not root:
return 0
if not root.left and not root.right:
return 1
left = getDepth(root.left)
right = getDepth(root.right)
if left==-1 or right == -1:
return -1
elif abs(left-right) > 1:
return -1
else:
return max(left, right) + 1
return getDepth(root) != -1