leetcode 133 Clone Graph
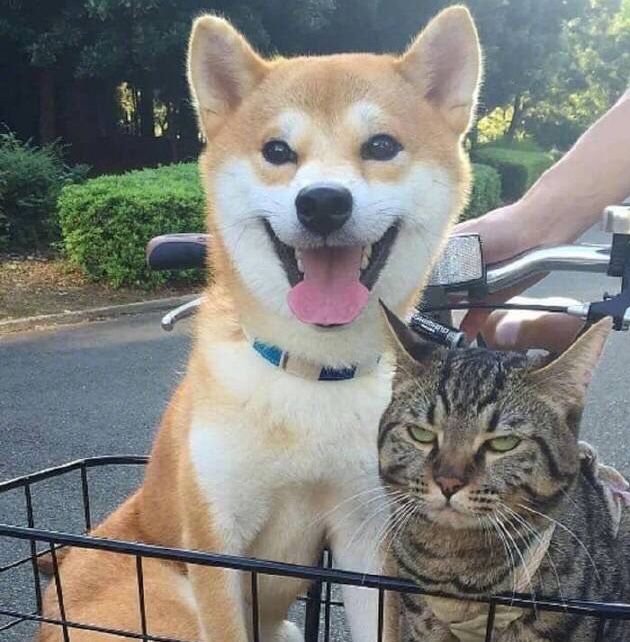
Given a reference of a node in a connected undirected graph, return a deep copy (clone) of the graph. Each node in the graph contains a val (int
) and a list (List[Node]
) of its neighbors.
Example:
1 | Input: |
Note:
- The number of nodes will be between 1 and 100.
- The undirected graph is a simple graph, which means no repeated edges and no self-loops in the graph.
- Since the graph is undirected, if node p has node q as neighbor, then node q must have node p as neighbor too.
- You must return the copy of the given node as a reference to the cloned graph.
使用一个map来保存源节点
Node
和与之对应的deep copy of Node
的对应关系。遍历
Node
的每一个neighbor
, 将如果neighbor
没有保存在map中,建立一个neighbor
和deep copy of neighbor
的对应关系。并将deep copy of neighbor
加入deep copy of Node
的neighbots
中。对
Node
的每一个neighbor
重复上述操作。需要注意的是,每次输入的
Node
有可能已经操作过了,因此,每次操作开始前,需要判断Node
是否已经处理过,即判断deep copy of Node
的neighbors
长度是否为0即可
1 | /* |