leetcode 236 Lowest Common Ancestor of a Binary Tree
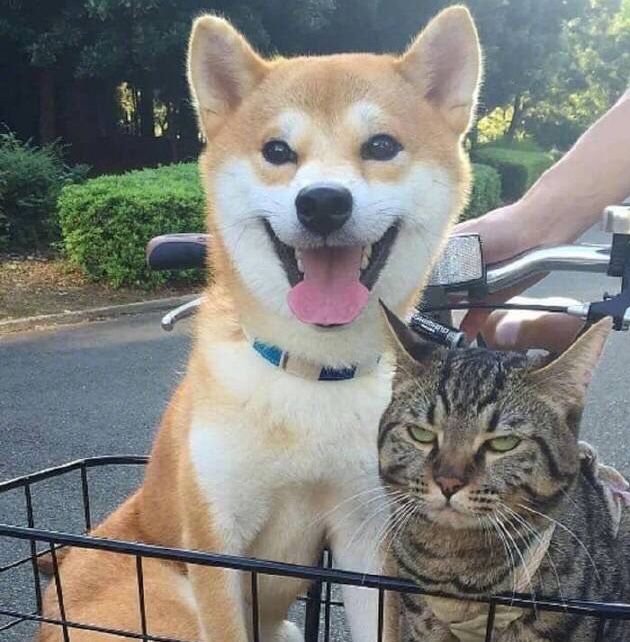
Given a binary tree, find the lowest common ancestor (LCA) of two given nodes in the tree.
According to the definition of LCA on Wikipedia: “The lowest common ancestor is defined between two nodes p and q as the lowest node in T that has both p and q as descendants (where we allow a node to be a descendant of itself).”
Given the following binary tree: root = [3,5,1,6,2,0,8,null,null,7,4]
Example 1:
1 | Input: root = [3,5,1,6,2,0,8,null,null,7,4], p = 5, q = 1 |
Example 2:
1 | Input: root = [3,5,1,6,2,0,8,null,null,7,4], p = 5, q = 4 |
Note:
- All of the nodes’ values will be unique.
- p and q are different and both values will exist in the binary tree.
- 最小公共节点的特征: p 和 q 要么分别来自它的左右分支,要么p和q中有一本身就是最小公共节点。
- 这样一来,可以用递归的方式,先依次遍历每一个节点。递归的在每一个节点的左右节点查找p和q
- 如果当前节点的左右分支都有返回值,那么当前节点就是一个最小公共节点
- 否则,由于公共最小节点可定存在,那么左右分支中必有一个是返回值。返回左右节点中非空的那个
1 | class Solution { |