leetcode 316 Remove Duplicate Letters
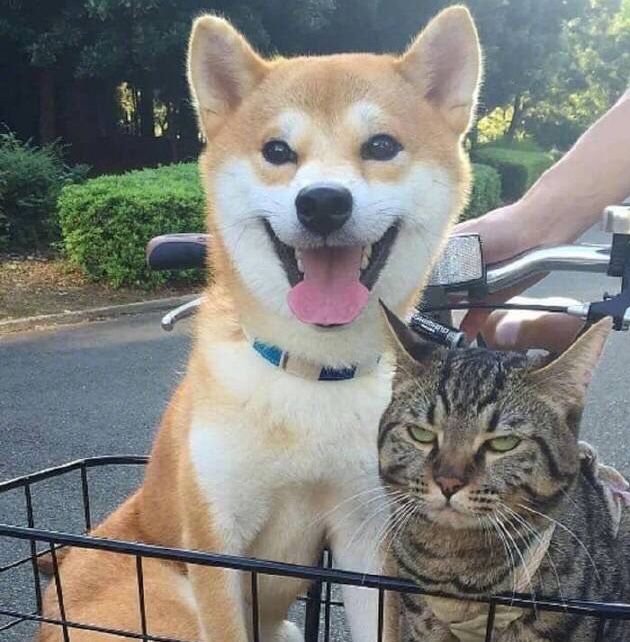
Given a string which contains only lowercase letters, remove duplicate letters so that every letter appear once and only once. You must make sure your result is the smallest in lexicographical order among all possible results.
Example 1:
1 | Input: "bcabc" |
Example 2:
1 | Input: "cbacdcbc" |
先用一个count数组保存每一个字符出现的次数。
然后从左到右遍历count数组,同时保存当前遇到的最小的字符的下表。
每遇到一个新的字符,将count数组中对应的位置减1,如果得到0,说明再往后就不再会出现该字符了,那么就需要把该字符之前的最小的字符保存到返回的string中。
1 | class Solution { |