leetcode 400 Nth Digit
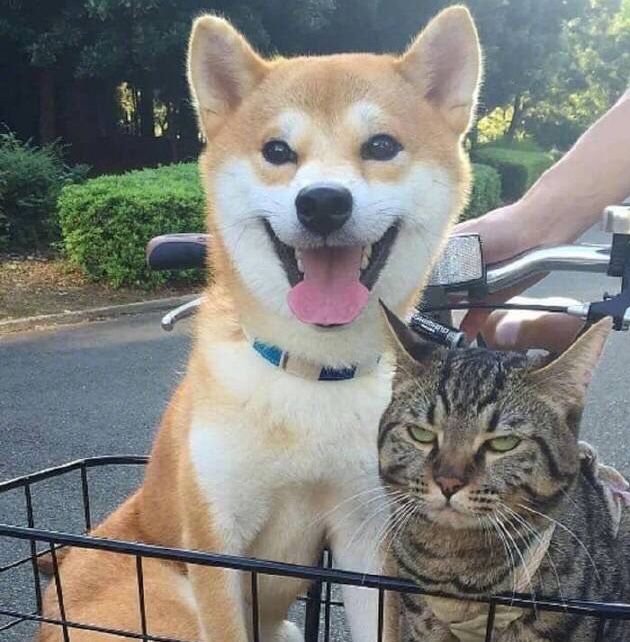
Find the $n$ th digit of the infinite integer sequence 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, …
Note:
n is positive and will fit within the range of a 32-bit signed integer (n < 231).
Example 1:
1 | Input: |
Example 2:
1 | Input: |
先总结一下规律
1
2
3
4
5
6
7
80 1 2 3 4 5 6 7 8 9 -> start = 0, width = 1, count = 9
---
10 11 12 13 14 15 16 17 18 19 -> start = 10, width = 2, count = 90
20 21 22 23 24 25 26 27 28 29
...
90 91 92 93 94 95 96 97 98 99
---
100 101 102 .... -> start = 100, width = 3, count = 900用
start
来保存每一个分组的开始的数组用
width
来保存每当前分组中,每一个数包涵几个数字用
count
来保存每一个分组中,一共有多少个数那么,每一个分组中,一共包含
count * width
位数第一步,先找到
n
位于哪个分组,找到该分组的start
第二步,找到
n
位于当前分组的那个数中第三步,确定
n
位是当前这数的第几个位
1 | class Solution { |