leetcode 410 Split Array Largest Sum
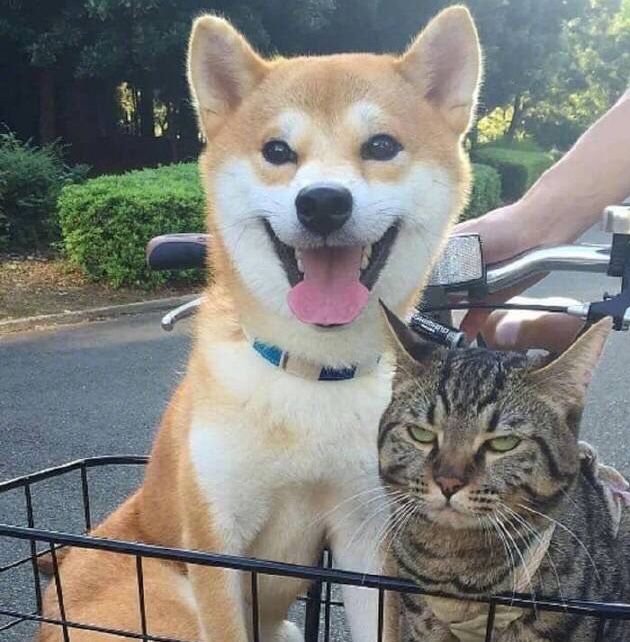
Given an array which consists of non-negative integers and an integer m, you can split the array into m non-empty continuous subarrays. Write an algorithm to minimize the largest sum among these msubarrays.
Note:
If n is the length of array, assume the following constraints are satisfied:
- 1 ≤ n ≤ 1000
- 1 ≤ m ≤ min(50, n)
Examples:
1 | Input: |
Binary Search
- 最小的返回值是,当
m
为len(nums)
的时候,max(nums)
就是返回值。 - 最大的返回值是,当
m
为1
的时候,sum(nums)
就是返回值。 - 因此,我们可以在
max(nums)
和sum(nums)
这个区间找到minimize largest sum
- 采用二分查找的方法,取中位数
mid
。判断中位数是否能够成为每一个split的larget sum - 遍历
nums
数组,贪心的进行分组。如果累加到当前位置的和大于mid
,就进行一个新的划分。并统计当前的划分个数。 - 如果划分个数已经大于
m
。说明还有多的数。那么minimize largest sum
应该大于mid
,这样,left = mid+1
。 - 否则,就说明当前的数都已经有了划分,并且要么划分个数没有到达
m
个,或者刚好等于m
个。- 如果是没有到达
m
个,就需要从其他的划分中取出数组成新的split,这样一来,当前的minimize largest sum
要进一步减小,right = mid
- 如果已经到达了
m
个,第m
个split中的sum
可能这m个split的每一个sum都小于mid
, 当前的minimize largest sum
要进一步缉拿小,right = mid
- 如果已经到达了
- 如果是没有到达
- 采用二分查找的方法,取中位数
1 | class Solution { |