leetcode 450 Delete Node in a BST
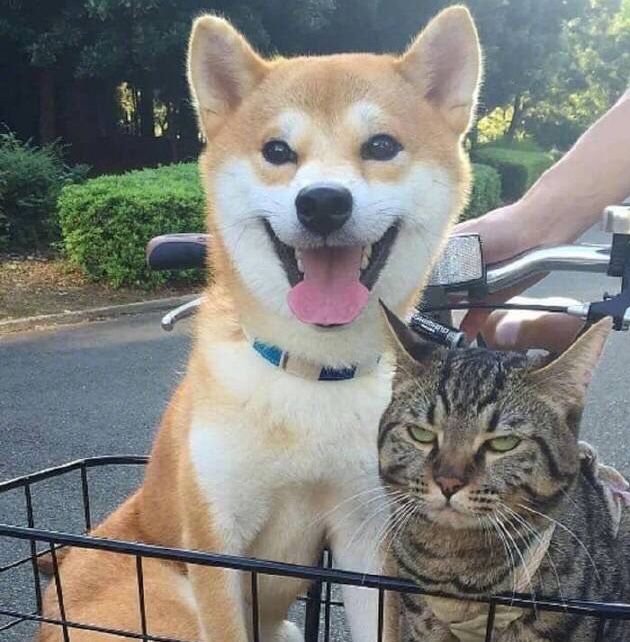
Given a root node reference of a BST and a key, delete the node with the given key in the BST. Return the root node reference (possibly updated) of the BST.
Basically, the deletion can be divided into two stages:
- Search for a node to remove.
- If the node is found, delete the node.
Note: Time complexity should be O(height of tree).
Example:
1 | root = [5,3,6,2,4,null,7] |
在一颗二叉搜索树中,去掉指定的数,构建新的二叉搜索树。
- 我们假设deleteNode函数返回已经修改的二叉搜索树的子树,我们递归的寻找需要删除的那个root
- 当我们找到了这个root之后,判断这个root是否只有一个左子树或者右子树,这种情况下,只要把当前的root替换为其左子树的root或者右子树的root即可
- 如果我们要delete的这个root有左右子树都有
- 那么我么去他的左子树,找到他左子树的最大的数,令root的值等于该最大值,并且在其root的左子树中delete该最大值节点
- 或者我们去他的右子树,找到他右子树的最小的数,令root的值等于该最小值,并且在其root的右子树中delete该最小节点
1 | # Definition for a binary tree node. |