leetcode 50 Pow(x, n)
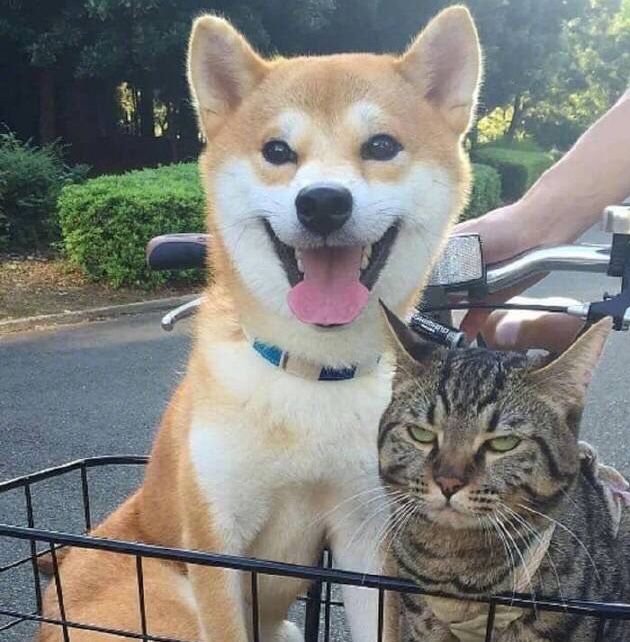
50. Pow(x, n)
Implement pow(x, n).
Example 1:
1 | Input: 2.00000, 10 |
Example 2:
1 | Input: 2.10000, 3 |
- 最要考察递归,初始的idea是
pow(x, n) = x*pow(x, n-1)
但是这样的递归势必会造成栈溢出 - 如果n是2的倍数,则可以进一步转换为
pow(x, n) = pow(x,n/2)*pow(x, n/2)
但是这样有包含重复计算的子问题 - 进一步转换
pow(x, n) = pow(x*x,n/2)
- 同时,考虑当
n
小于0时,$x^n = \frac{1}{x}^{-n}$ 所以我们需要将x = 1/x, 同时 n = -n; - 注意: 在对一个int类型取反的时候,一定要考虑溢出问题,对于一个int类型的数,范围为[$-2^{31}, 2^{31}-1$], 如果对$-2^{31}$ 取反,就会造成溢出问题,因此需要考虑当
n== Integer.MIN_VALUE
的问题。 - 当
n== Integer.MIN_VALUE
,如果x==1的话,1的任何次方都为1,当x != 1 的话,相当于是$\frac{1}{x}^{+∞}$ == 0
1 | class Solution { |
1 |