leetcode 167 Two Sum II - Input array is sorted
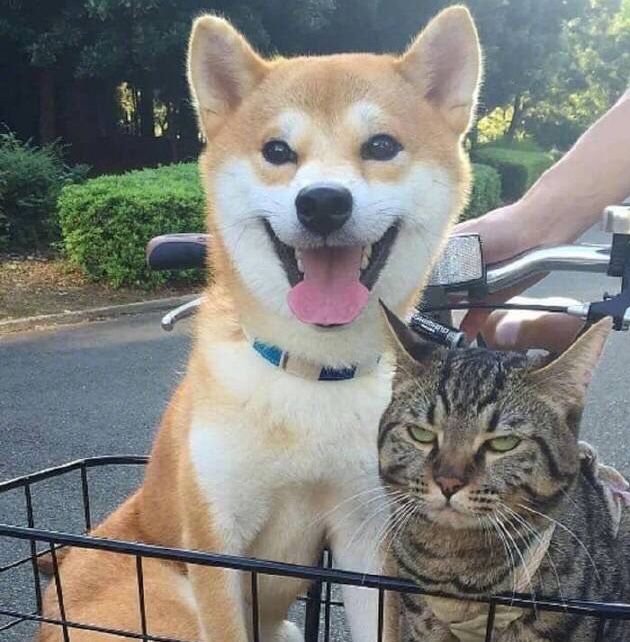
167. Two Sum II - Input array is sorted
Given an array of integers that is already sorted in ascending order, find two numbers such that they add up to a specific target number.
The function twoSum should return indices of the two numbers such that they add up to the target, where index1 must be less than index2. Please note that your returned answers (both index1 and index2) are not zero-based.
You may assume that each input would have exactly one solution and you may not use the same element twice.
Input: numbers={2, 7, 11, 15}, target=9
Output: index1=1, index2=2
第一想到的方法是之前two sum 1中的方法,使用一个hashmap
1 | class Solution { |
但是这里考虑到输入已经排序,而且一定存在一个解,那么可以直接比较:
1 | class Solution { |