leetcode 57 Insert Interval
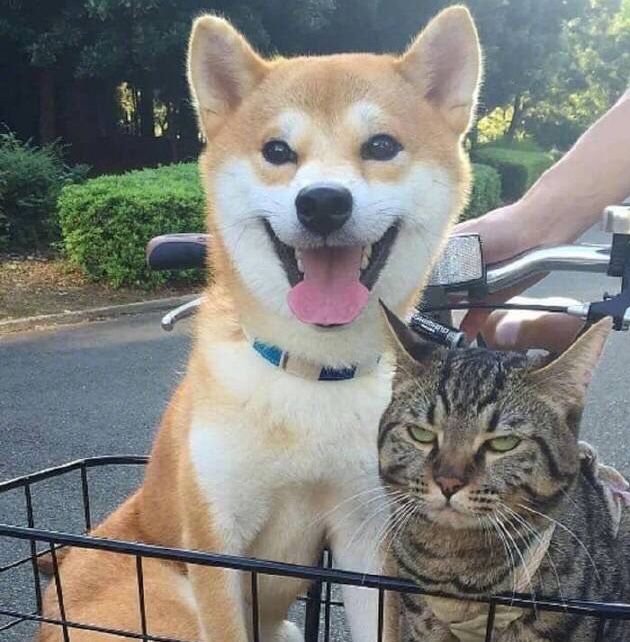
Given a set of non-overlapping intervals, insert a new interval into the intervals (merge if necessary).
You may assume that the intervals were initially sorted according to their start times.
Example 1:
1 | Input: intervals = [[1,3],[6,9]], newInterval = [2,5] |
Example 2:
1 | Input: intervals = [[1,2],[3,5],[6,7],[8,10],[12,16]], newInterval = [4,8] |
- 若
interval
在当前区间的右边,将当前区间添加到ans中 - 若
interval
在当前区间的左边,判断是否已经插入过interval
- 若没插入过
interval
,插入interval
,修改插入标记,插入当前区域 - 若已插入过
interval
,插入当前区域
- 若没插入过
- 若
interval
和当前区间有交集,将interval.start
取interval
和当前区间的最小值,interval.end
取interval和当前区域的最大值,继续循环。 - 循环结束,判断是否已经插入,若没插入,即将interval插入ans,结束。
1 |
|