leetcode 88 Merge Sorted Array
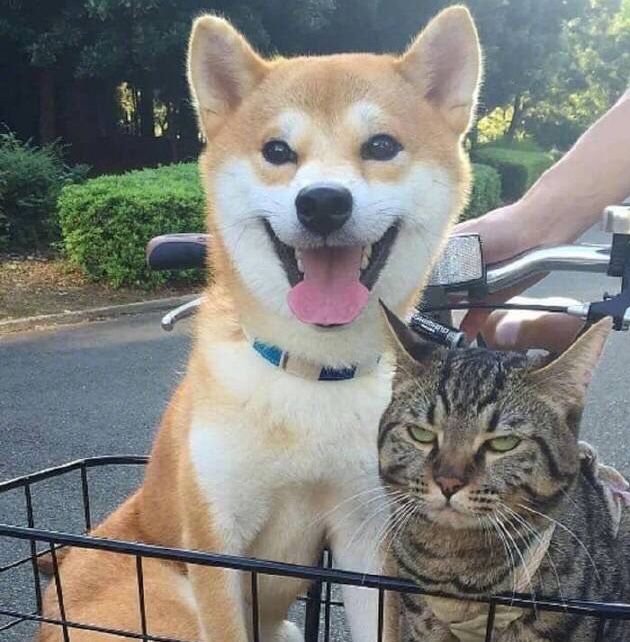
88. Merge Sorted Array
Given two sorted integer arrays nums1 and nums2, merge nums2 into nums1 as one sorted array.
Note:
You may assume that nums1 has enough space (size that is greater or equal to m + n) to hold additional elements from nums2. The number of elements initialized in nums1 and nums2 are m and n respectively.
最初始的想法是从前往后遍历,找到一个nums1[i] > nums2[j],把nums1从i开始后移一位。但是这样的时间复杂度较高
再然后适用一个新的数组result[m+n],时间复杂度为O(m+n),但是空间复杂度也有O(m+n)
再考虑从后往前遍历两个数组,时间复杂度为O(m+n), 空间复杂度为O(1).
1 | class Solution { |