leetcode solution 96 Unique Binary Search Trees
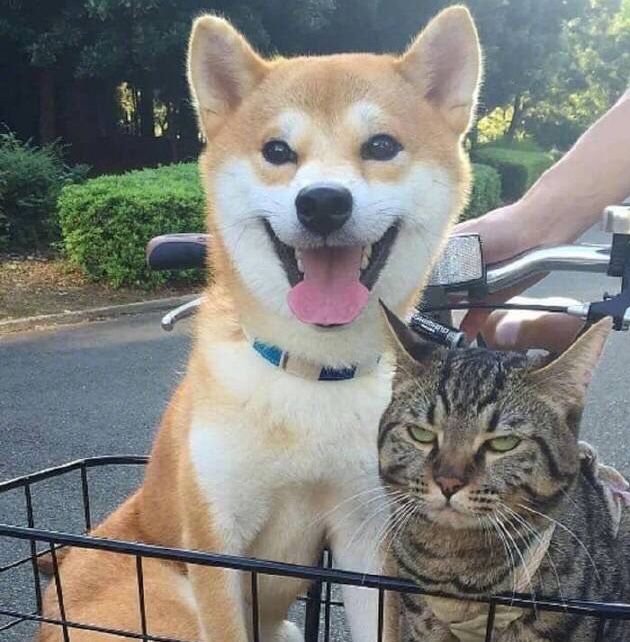
Given n, how many structurally unique BST’s (binary search trees) that store values 1…n?
For example,
Given n = 3, there are a total of 5 unique BST’s.
1 | 1 3 3 2 1 |
1 | class Solution { |
- 对于n = 5
- 1 2 3 4 5
- dp[0] = 1
- dp[1] = 1
- dp[2] =当1为根节点和当2为更节点之和
- dp[4]
- 1为根节点 + 2为根节点 + 3为根结点 + 3为根节点
- 当j为根节点时, 可能的组合为 左子树的可能组合*右子树的可能组合
- 假设跟个节点为2, 左子树
1
右子树3 4
,而3 4
等价于1 2